Chapter 3 - Making money
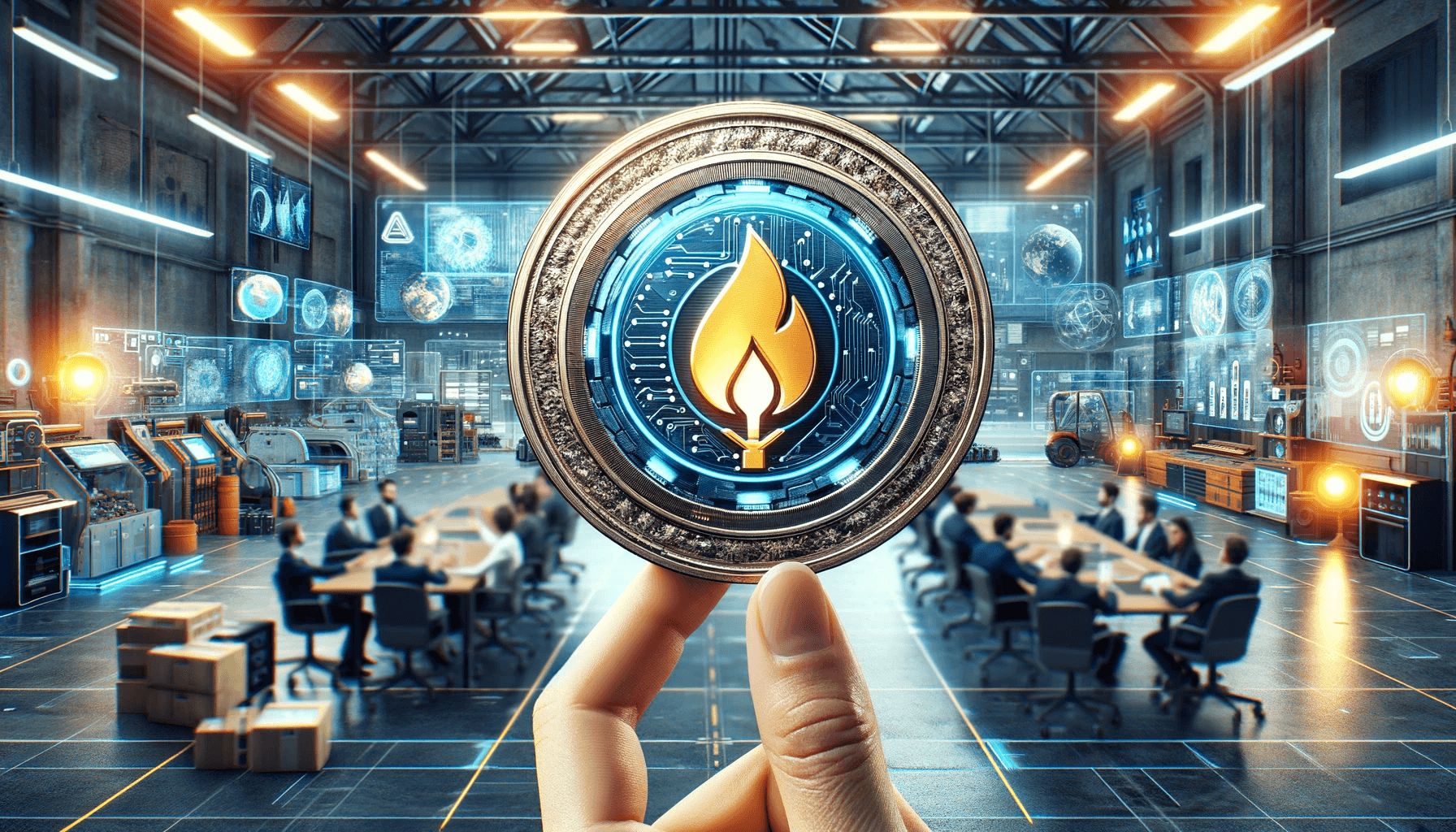
In this chapter we implement a token for our DAO. This is an essential step to make our project economically sustainable. A mistake commonly made when creating a DAO is to believe that having a token is enough to guarantee a solid business strategy and consistent income for the organization - this is not the case.
Introduction
Creating and distributing a token is an essential step in any DAO. A token enables the creation of a market, enabling investors to invest in the DAO, community members to receive rewards for their contributions, and usually facilitates governance by allowing token holders to vote on key decisions and initiatives. Today, your mission, is to implement the code for a token.
On the Internet Computer, we can create a token by creating a canister that stores balances and manage transfer. Assuming the canister is not controlled we can consider the token to be safe, trustless and decentralized. In this project you will implement the code for a simple token. If you are interested in learning more about tokens, you can read the ICRC_1 standard.
Resources
To complete this Chapter, we suggest browsing the following resources:
Tasks
- Define the
ledger
variable. This variable will be used to store the balance of each user. The keys are of typePrincipal
and the values of typeNat
. You can use theHashMap
orTrieMap
types to implement this variable. - Implement the
tokenName
function, this function takes no parameters and returns the name of your token as aText
.
Choose any name you want for your token.
- Implement the
tokenSymbol
function, this function takes no parameters and returns the symbol of your token as aText
.
Choose a symbol that is exactly 3 characters in length.
- Implement the
mint
function. This function takes aPrincipal
and aNat
as arguments. It adds theNat
to the balance of the givenPrincipal
. You will use theResult
type for your return value. - Implement the
burn
function. This function takes aPrincipal
and aNat
as arguments. It subtracts theNat
from the balance of the givenPrincipal
. You will use theResult
type for your return value. - Implement the
transfer
function. This function takes aPrincipal
representing the sender (from), aPrincipal
representing the recipient (to), and aNat
value for the amount to be transferred. It transfers the specified amount of tokens from the sender's account to the recipient's account. You will use theResult
type for your return value. - Implement the
balanceOf
query function. This function takes aPrincipal
as an argument and returns the balance of the given account as aNat
. It should return 0 if the account does not exist in theledger
variable. - Implement the
totalSupply
query function. This function takes no parameters and returns the total supply of your token as aNat
. The total supply is calculated by adding together the balances of every user stored in theledger
variable. - Complete Chapter 3 by deploying your canister and submitting your ID on motokobootcamp.com.
To deploy your application run
dfx deploy --playground chapter_3
.
Video
⚠️ Please be aware: the repository displayed in the video may not match the one you're working with, due to recent updates we've made to the repository which have not been reflected in the video. However, the core code should remain similar.